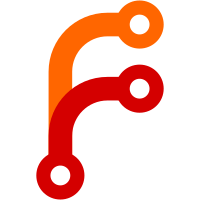
The primary license of the project is changing to: AGPL-3.0-or-later WITH Web-Template-Output-Additional-Permission-3.0-or-later with some specific files to be licensed under the one of two licenses: CC0-1.0 LGPL-3.0-or-later This commit is one of the many steps to relicense the entire codebase. Documentation granting permission for this relicensing (from all past contributors who hold copyrights) is on file with Software Freedom Conservancy, Inc.
47 lines
1.3 KiB
Ruby
47 lines
1.3 KiB
Ruby
# License: AGPL-3.0-or-later WITH Web-Template-Output-Additional-Permission-3.0-or-later
|
|
# For tracking and calculating timespans/time intervals
|
|
# Relies on activesupport
|
|
|
|
Timespan = Struct.new(:interval, :time_unit) do
|
|
|
|
Units = ['week', 'day', 'month', 'year']
|
|
TimeUnits = {
|
|
'1_week' => 1.week.ago,
|
|
'2_weeks' => 2.weeks.ago,
|
|
'1_month' => 1.month.ago,
|
|
'3_months' => 3.months.ago,
|
|
'6_months' => 6.months.ago,
|
|
'1_year' => 1.year.ago,
|
|
'2_years' => 2.years.ago
|
|
}
|
|
|
|
# Test if end_date is past start_date by timespan
|
|
# eg: later_than_by?(Jun 13th, Jul 14th, 1.month) -> true
|
|
# Special case:
|
|
# later_than_by?(Jan 31st, Feb 28th, 1.month) -> true
|
|
def self.later_than_by?(start_date, end_date, timespan)
|
|
return (start_date + timespan) <= end_date
|
|
end
|
|
|
|
# Given an Integer (frequency) and a String (time unit),
|
|
# return the timespan object (ie. number of seconds) constituting the timespan
|
|
# timespan(1, 'minute') -> 60
|
|
# timespan(1, 'month') -> 2592000
|
|
def self.create(interval, time_unit)
|
|
raise(ArgumentError, "time_unit must be one of: #{Units}") unless Units.include?(time_unit)
|
|
return interval.send(time_unit.to_sym)
|
|
end
|
|
|
|
def self.in_future?(datetime)
|
|
datetime > Time.current
|
|
end
|
|
|
|
def self.date_now_or_in_future?(date)
|
|
date >= Date.today
|
|
end
|
|
|
|
def self.in_past?(date)
|
|
date < Time.current
|
|
end
|
|
|
|
end
|