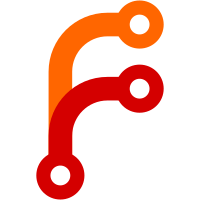
The primary license of the project is changing to: AGPL-3.0-or-later WITH Web-Template-Output-Additional-Permission-3.0-or-later The Additional Permission is designed to permit publicly distributed Javascript code to be relicensed under LGPL-3.0-or-later, but not server-side Javascript code. As such, we've relicensed here static Javscript files under LGPL-3.0-or-later, and those that run as part of build and/or server side under AGPL-3.0-or-later. Note that in future, Javascript files may be updated to be stronger copyleft license with the Additional Permission, particularly if they adapted to run on server side and/or turned into templates. Of course, we'd seek public discussion with the contributor community about such changes. This commit is one of the many steps to relicense the entire codebase. Documentation granting permission for this relicensing (from all past contributors who hold copyrights) is on file with Software Freedom Conservancy, Inc.
114 lines
4.2 KiB
JavaScript
114 lines
4.2 KiB
JavaScript
// License: LGPL-3.0-or-later
|
|
const snabbdom = require('snabbdom')
|
|
const flyd = require('flyd')
|
|
const render = require('ff-core/render')
|
|
const amount = require("../../../../client/js/nonprofits/donate/amount-step")
|
|
const R = require('ramda')
|
|
const assert = require('assert')
|
|
|
|
window.log = x => y => console.log(x,y)
|
|
window.app = {
|
|
nonprofit: {
|
|
id: 1
|
|
, name: 'test npo'
|
|
, logo: { normal: {url: 'xyz.com'} }
|
|
, tagline: 'whasup'
|
|
}
|
|
}
|
|
|
|
const patch = snabbdom.init([
|
|
require('snabbdom/modules/eventlisteners')
|
|
, require('snabbdom/modules/class')
|
|
, require('snabbdom/modules/props')
|
|
, require('snabbdom/modules/style')
|
|
])
|
|
|
|
const init = (donationDefaults, params$) => {
|
|
let div = document.createElement('div')
|
|
let state = amount.init(donationDefaults||{}, params$||flyd.stream({}))
|
|
let streams = render({
|
|
container: div
|
|
, state: state
|
|
, patch: patch
|
|
, view: amount.view
|
|
})
|
|
streams.state = state
|
|
return streams
|
|
}
|
|
|
|
const allText = R.map(R.prop('textContent'))
|
|
const defaultDesigOptions = ['Choose a designation (optional)', 'Use my donation where most needed']
|
|
|
|
suite("donate wiz / amount step")
|
|
test("shows a designation dropdown if the multiple_designations param is set", ()=> {
|
|
let streams = init({}, flyd.stream({multiple_designations: ['a','b']}))
|
|
let options = allText(streams.dom$().querySelectorAll('.donate-designationDropdown option'))
|
|
assert.deepEqual(options, R.concat(defaultDesigOptions, ['a', 'b']))
|
|
})
|
|
|
|
test('sets no designation with a dropdown on the default value', () => {
|
|
let streams = init({}, flyd.stream({multiple_designations: ['a', 'b']}))
|
|
let change = document.createEvent('Event')
|
|
change.initEvent('change', false, false, null )
|
|
let select = streams.dom$().querySelector('.donate-designationDropdown')
|
|
select.selectedIndex = 0
|
|
select.dispatchEvent(change)
|
|
assert.equal(streams.state.donation$().designation, '')
|
|
select.selectedIndex = 1
|
|
select.dispatchEvent(change)
|
|
assert.equal(streams.state.donation$().designation, '')
|
|
})
|
|
|
|
test("changing the dropdown sets the designation", () => {
|
|
let streams = init({}, flyd.stream({multiple_designations: ['a', 'b']}))
|
|
let change = document.createEvent('Event')
|
|
change.initEvent('change', false, false, null )
|
|
let select = streams.dom$().querySelector('.donate-designationDropdown')
|
|
select.selectedIndex = 2
|
|
select.dispatchEvent(change)
|
|
assert.equal(streams.state.donation$().designation, 'a')
|
|
})
|
|
|
|
test("shows no dropdown if the multiple_designations param is not set", ()=> {
|
|
let streams = init()
|
|
let drop = streams.dom$().querySelector('.donate-designationDropdown')
|
|
assert.equal(drop, null)
|
|
})
|
|
|
|
test("shows a recurring donation checkbox by default", ()=> {
|
|
let streams = init()
|
|
assert(streams.dom$().querySelector('.donate-recurringCheckbox'))
|
|
})
|
|
|
|
test("hides the recurring donation checkbox if params type is set to recurring", ()=> {
|
|
let streams = init({}, flyd.stream({type: 'recurring'}))
|
|
let check = streams.dom$().querySelector('.donate-recurringCheckbox')
|
|
assert.equal(check, null)
|
|
})
|
|
|
|
test("shows a recurring message if the recurring box is checked", ()=> {
|
|
let streams = init()
|
|
let change = document.createEvent('Event')
|
|
change.initEvent('change', false, false, null )
|
|
streams.dom$().querySelector('.donate-recurringCheckbox input').dispatchEvent(change)
|
|
const msg = streams.dom$().querySelector('.donate-recurringMessage').textContent
|
|
assert.equal(msg, 'Select an amount for your monthly contribution')
|
|
})
|
|
|
|
test("shows a recurring message if the type in params is set to recurring", ()=> {
|
|
let streams = init({}, flyd.stream({type: 'recurring'}))
|
|
const msg = streams.dom$().querySelector('.donate-recurringMessage').textContent
|
|
assert.equal(msg, 'Select an amount for your monthly contribution')
|
|
})
|
|
|
|
test("does not show a recurring message if the type is one-time in params", ()=> {
|
|
let streams = init({}, flyd.stream({type: 'one-time'}))
|
|
const msg = streams.dom$().querySelector('.donate-recurringMessage')
|
|
assert.equal(msg, null)
|
|
})
|
|
|
|
test("does not show a recurring message if the type is one-time in params", ()=> {
|
|
let streams = init({}, flyd.stream({type: 'one-time'}))
|
|
const msg = streams.dom$().querySelector('.donate-recurringCheckbox')
|
|
assert.equal(msg, null)
|
|
})
|