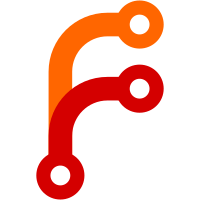
The main impetus of this change is to rename accounts that were outside Beancount's accepted five root accounts, to move them into that structure. This includes: Accrued:*Payable: → Liabilities:Payable:* Accrued:*Receivable: → Assets:Receivable:* UneanedIncome:* → Liabilities:UnearnedIncome:* Note the last change did inspire in a change to our validation rules. We no longer require income-type on unearned income, because it's no longer considered income at all. Once it's earned and converted to an Income account, that has an income-type of course. This did inspire another rename that was not required, but provided more consistency with the other account names above: Assets:Prepaid* → Assets:Prepaid:* Where applicable, I have generally extended tests to make sure one of each of the five account types is tested. (This mostly meant adding an Equity account to the tests.) I also added tests for key parts of the hierarchy, like Assets:Receivable and Liabilities:Payable, where applicable. As part of this change, Account.is_real_asset() got renamed to Account.is_cash_equivalent(), to better self-document its purpose.
134 lines
4.4 KiB
Python
134 lines
4.4 KiB
Python
"""Test handling of expense-allocation metadata"""
|
|
# Copyright © 2020 Brett Smith
|
|
#
|
|
# This program is free software: you can redistribute it and/or modify
|
|
# it under the terms of the GNU Affero General Public License as published by
|
|
# the Free Software Foundation, either version 3 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU Affero General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU Affero General Public License
|
|
# along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
|
|
import pytest
|
|
|
|
from . import testutil
|
|
|
|
from conservancy_beancount.plugin import meta_expense_allocation
|
|
|
|
VALID_VALUES = {
|
|
'program': 'program',
|
|
'administration': 'administration',
|
|
'fundraising': 'fundraising',
|
|
'admin': 'administration',
|
|
}
|
|
|
|
INVALID_VALUES = {
|
|
'invalid',
|
|
'porgram',
|
|
'adimn',
|
|
'fundrasing',
|
|
'',
|
|
}
|
|
|
|
TEST_KEY = 'expense-allocation'
|
|
|
|
@pytest.fixture(scope='module')
|
|
def hook():
|
|
config = testutil.TestConfig()
|
|
return meta_expense_allocation.MetaExpenseAllocation(config)
|
|
|
|
@pytest.mark.parametrize('src_value,set_value', VALID_VALUES.items())
|
|
def test_valid_values_on_postings(hook, src_value, set_value):
|
|
txn = testutil.Transaction(postings=[
|
|
('Assets:Cash', -25),
|
|
('Expenses:General', 25, {TEST_KEY: src_value}),
|
|
])
|
|
errors = list(hook.run(txn))
|
|
assert not errors
|
|
testutil.check_post_meta(txn, None, {TEST_KEY: set_value})
|
|
|
|
@pytest.mark.parametrize('src_value', INVALID_VALUES)
|
|
def test_invalid_values_on_postings(hook, src_value):
|
|
txn = testutil.Transaction(postings=[
|
|
('Assets:Cash', -25),
|
|
('Expenses:General', 25, {TEST_KEY: src_value}),
|
|
])
|
|
errors = list(hook.run(txn))
|
|
assert errors
|
|
testutil.check_post_meta(txn, None, {TEST_KEY: src_value})
|
|
|
|
@pytest.mark.parametrize('src_value,set_value', VALID_VALUES.items())
|
|
def test_valid_values_on_transactions(hook, src_value, set_value):
|
|
txn = testutil.Transaction(**{TEST_KEY: src_value}, postings=[
|
|
('Assets:Cash', -25),
|
|
('Expenses:General', 25),
|
|
])
|
|
errors = list(hook.run(txn))
|
|
assert not errors
|
|
testutil.check_post_meta(txn, None, {TEST_KEY: set_value})
|
|
|
|
@pytest.mark.parametrize('src_value', INVALID_VALUES)
|
|
def test_invalid_values_on_transactions(hook, src_value):
|
|
txn = testutil.Transaction(**{TEST_KEY: src_value}, postings=[
|
|
('Assets:Cash', -25),
|
|
('Expenses:General', 25),
|
|
])
|
|
errors = list(hook.run(txn))
|
|
assert errors
|
|
testutil.check_post_meta(txn, None, None)
|
|
|
|
@pytest.mark.parametrize('account', [
|
|
'Assets:Cash',
|
|
'Assets:Receivable:Accounts',
|
|
'Equity:OpeningBalance',
|
|
'Income:Other',
|
|
'Liabilities:CreditCard',
|
|
'Liabilities:Payable:Vacation',
|
|
])
|
|
def test_non_expense_accounts_skipped(hook, account):
|
|
meta = {TEST_KEY: 'program'}
|
|
txn = testutil.Transaction(postings=[
|
|
(account, -25),
|
|
('Expenses:General', 25, meta.copy()),
|
|
])
|
|
errors = list(hook.run(txn))
|
|
assert not errors
|
|
testutil.check_post_meta(txn, None, meta)
|
|
|
|
@pytest.mark.parametrize('account,set_value', [
|
|
('Expenses:Services:Accounting', 'administration'),
|
|
('Expenses:Services:Administration', 'administration'),
|
|
('Expenses:Services:Advocacy', 'program'),
|
|
('Expenses:Services:Development', 'program'),
|
|
('Expenses:Services:Fundraising', 'fundraising'),
|
|
])
|
|
def test_default_values(hook, account, set_value):
|
|
txn = testutil.Transaction(postings=[
|
|
('Liabilites:CreditCard', -25),
|
|
(account, 25),
|
|
])
|
|
errors = list(hook.run(txn))
|
|
assert not errors
|
|
testutil.check_post_meta(txn, None, {TEST_KEY: set_value})
|
|
|
|
@pytest.mark.parametrize('date,set_value', [
|
|
(testutil.EXTREME_FUTURE_DATE, None),
|
|
(testutil.FUTURE_DATE, 'program'),
|
|
(testutil.FY_START_DATE, 'program'),
|
|
(testutil.FY_MID_DATE, 'program'),
|
|
(testutil.PAST_DATE, None),
|
|
])
|
|
def test_default_value_set_in_date_range(hook, date, set_value):
|
|
txn = testutil.Transaction(date=date, postings=[
|
|
('Liabilites:CreditCard', -25),
|
|
('Expenses:General', 25),
|
|
])
|
|
errors = list(hook.run(txn))
|
|
assert not errors
|
|
expect_meta = None if set_value is None else {TEST_KEY: set_value}
|
|
testutil.check_post_meta(txn, None, expect_meta)
|