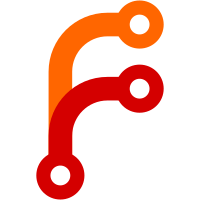
The format option on bean-query command-line is now an option that the client can pass along. The setting defaults to whatever `bean-query` was going to default to.
44 lines
1.6 KiB
Perl
44 lines
1.6 KiB
Perl
# License: AGPLv3-or-later
|
|
# see files COPYRIGHT, LICENSE, and AGPL-3.0.txt included in canonical repository for details.
|
|
# https://k.sfconservancy.org/NPO-Accounting/beancount-tools-in-Perl
|
|
|
|
use strict;
|
|
use warnings;
|
|
|
|
our %BEANCOUNT_QUERY;
|
|
|
|
my $IPC_GLUE = 'BeAn';
|
|
|
|
sub BeancountQueryInitialize {
|
|
my %options = (create => 0, exclusive => 0, mode => 0600, destroy => 0);
|
|
tie %BEANCOUNT_QUERY, 'IPC::Shareable', $IPC_GLUE, { %options } or
|
|
die "BEANCOUNT_QUERY: tie failed: is the goffy beancount server running?\n";
|
|
}
|
|
|
|
sub BeancountQuerySubmit($;$) {
|
|
my($question, $format) = @_;
|
|
while (defined $BEANCOUNT_QUERY{fifoName} or defined $BEANCOUNT_QUERY{question}) { sleep 1; }
|
|
(tied %BEANCOUNT_QUERY)->shlock;
|
|
if (defined $BEANCOUNT_QUERY{fifoName} or defined $BEANCOUNT_QUERY{question}) {
|
|
(tied %BEANCOUNT_QUERY)->shunlock;
|
|
no warnings 'uninitialized';
|
|
die("caught lock to submit a query, but either fifoName or question was defined, " .
|
|
"so something is wrong here. " .
|
|
"fifoName: \"$BEANCOUNT_QUERY{fifoName}\" question: \"$BEANCOUNT_QUERY{question}\"!");
|
|
}
|
|
$BEANCOUNT_QUERY{question} = $question;
|
|
$BEANCOUNT_QUERY{format} = $format if defined $format;
|
|
(tied %BEANCOUNT_QUERY)->shunlock;
|
|
while (not defined $BEANCOUNT_QUERY{fifoName}) { sleep 1; }
|
|
die "Ceci n'est pas une pipe: BEANCOUNT_QUERY{fifoName}, $BEANCOUNT_QUERY{fifoName}:$!"
|
|
unless (-p $BEANCOUNT_QUERY{fifoName});
|
|
(tied %BEANCOUNT_QUERY)->shlock;
|
|
return $BEANCOUNT_QUERY{fifoName};
|
|
}
|
|
|
|
sub BeancountQueryComplete {
|
|
$BEANCOUNT_QUERY{question} = undef;
|
|
(tied %BEANCOUNT_QUERY)->shunlock;
|
|
}
|
|
|
|
1;
|