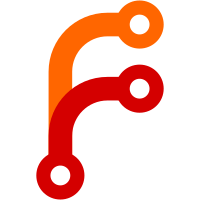
Bulk action marks printed + schwag, but as print is automated and happens after checkin is flagged, we should only set checkin and schwag and print will happen.
143 lines
4.8 KiB
Python
143 lines
4.8 KiB
Python
# -*- coding: utf-8 -*-
|
|
import base64
|
|
from datetime import datetime
|
|
from decimal import Decimal
|
|
from io import BytesIO
|
|
|
|
from django.core.exceptions import ValidationError
|
|
from django.utils import timezone
|
|
|
|
from django.db import models
|
|
from django.db.models import Q, F
|
|
from django.db.models import Case, When, Value
|
|
from django.db.models import Count
|
|
from django.db.models.signals import post_save
|
|
from django.contrib.auth.models import User
|
|
import pyqrcode
|
|
|
|
from symposion import constants
|
|
from symposion.text_parser import parse
|
|
from registrasion.models import commerce
|
|
from registrasion.util import generate_access_code as generate_code
|
|
|
|
|
|
class BoardingPassTemplate(models.Model):
|
|
|
|
label = models.CharField(max_length=100, verbose_name="Label")
|
|
from_address = models.EmailField(verbose_name="From address")
|
|
subject = models.CharField(max_length=100, verbose_name="Subject")
|
|
body = models.TextField(verbose_name="Body")
|
|
html_body = models.TextField(verbose_name="HTML Body",null=True)
|
|
|
|
class Meta:
|
|
verbose_name = ("Boarding Pass template")
|
|
verbose_name_plural = ("Boarding Pass templates")
|
|
|
|
class BoardingPass(models.Model):
|
|
|
|
template = models.ForeignKey(BoardingPassTemplate, null=True, blank=True,
|
|
on_delete=models.SET_NULL, verbose_name="Template")
|
|
created = models.DateTimeField(auto_now_add=True, verbose_name="Created")
|
|
sent = models.DateTimeField(null=True, verbose_name="Sent")
|
|
to_address = models.EmailField(verbose_name="To address")
|
|
from_address = models.EmailField(verbose_name="From address")
|
|
subject = models.CharField(max_length=255, verbose_name="Subject")
|
|
body = models.TextField(verbose_name="Body")
|
|
html_body = models.TextField(verbose_name="HTML Body", null=True)
|
|
|
|
class Meta:
|
|
permissions = (
|
|
("view_boarding_pass", "Can view sent boarding passes"),
|
|
("send_boarding_pass", "Can send boarding passes"),
|
|
)
|
|
|
|
def __unicode__(self):
|
|
return self.checkin.attendee.attendeeprofilebase.attendeeprofile.name + ' ' + self.timestamp.strftime('%Y-%m-%d %H:%M:%S')
|
|
|
|
@property
|
|
def email_args(self):
|
|
return (self.subject, self.body, self.from_address, self.user.email)
|
|
|
|
class CheckIn(models.Model):
|
|
|
|
user = models.OneToOneField(User)
|
|
boardingpass = models.OneToOneField(BoardingPass, null=True,
|
|
blank=True, on_delete=models.SET_NULL)
|
|
seen = models.DateTimeField(null=True,blank=True)
|
|
checked_in = models.DateTimeField(null=True,blank=True)
|
|
checkin_code = models.CharField(
|
|
max_length=6,
|
|
unique=True,
|
|
db_index=True,
|
|
)
|
|
_checkin_code_png=models.TextField(max_length=512,null=True,blank=True)
|
|
badge_printed = models.BooleanField(default=False)
|
|
schwag_given = models.BooleanField(default=False)
|
|
checked_in_bool = models.BooleanField(default=False)
|
|
needs_review = models.BooleanField(default=False)
|
|
review_text = models.TextField(blank=True)
|
|
|
|
class Meta:
|
|
permissions = (
|
|
("view_checkin_details", "Can view the details of other user's checkins"),
|
|
)
|
|
|
|
def save(self, *a, **k):
|
|
while not self.checkin_code:
|
|
checkin_code = generate_code()
|
|
if CheckIn.objects.filter(checkin_code=checkin_code).count() == 0:
|
|
self.checkin_code = checkin_code
|
|
return super(CheckIn, self).save(*a, **k)
|
|
|
|
def mark_checked_in(self):
|
|
self.checked_in_bool = True
|
|
self.checked_in = timezone.now()
|
|
self.save()
|
|
|
|
def mark_badge_printed(self):
|
|
self.badge_printed = True
|
|
self.save()
|
|
|
|
def unset_badge(self):
|
|
self.badge_printed = False
|
|
self.save()
|
|
|
|
def mark_schwag_given(self):
|
|
self.schwag_given = True
|
|
self.save()
|
|
|
|
def bulk_mark_given(self):
|
|
self.checked_in_bool = True
|
|
self.schwag_given = True
|
|
self.save()
|
|
|
|
def set_exception(self, text):
|
|
self.needs_review = True
|
|
self.review_text = text
|
|
self.save()
|
|
|
|
@property
|
|
def code(self):
|
|
return self.checkin_code
|
|
|
|
@property
|
|
def qrcode(self):
|
|
"""Returns the QR Code for this checkin's code.
|
|
|
|
If this is the first time the QR code has been generated, cache it on the object.
|
|
If a code has already been cached, serve that.
|
|
|
|
Returns the raw PNG blob, unless b64=True, in which case the return value
|
|
is the base64encoded PNG blob."""
|
|
|
|
if not self.code:
|
|
return None
|
|
if not self._checkin_code_png:
|
|
qrcode = pyqrcode.create(self.code)
|
|
qr_io = BytesIO()
|
|
qrcode.png(qr_io, scale=6)
|
|
qr_io.seek(0)
|
|
self._checkin_code_png = base64.b64encode(qr_io.read()).decode('UTF-8')
|
|
self.save()
|
|
|
|
return self._checkin_code_png
|