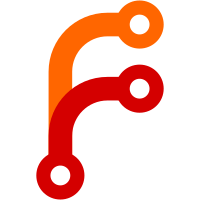
Upgrade site and modules to Django 2.2. Remove and replace obsolete functionality with current equivalents. Update requirements to latest versions where possible. Remove unused dependencies.
80 lines
2.3 KiB
Python
80 lines
2.3 KiB
Python
from functools import wraps
|
|
|
|
|
|
class MonkeyPatchMiddleware(object):
|
|
''' Ensures that our monkey patching only gets called after it is safe to do so.'''
|
|
|
|
def __init__(self, get_response):
|
|
self.get_response = get_response
|
|
|
|
def __call__(self, request):
|
|
do_monkey_patch()
|
|
response = self.get_response(request)
|
|
return response
|
|
|
|
|
|
def do_monkey_patch():
|
|
patch_stripe_payment_form()
|
|
|
|
# Remove this function from existence
|
|
global do_monkey_patch
|
|
do_monkey_patch = lambda: None # noqa: E731
|
|
|
|
|
|
def patch_stripe_payment_form(): # noqa: C901
|
|
|
|
import inspect # Oh no.
|
|
from django.http.request import HttpRequest
|
|
from registripe.forms import CreditCardForm
|
|
from pinaxcon.registrasion import models
|
|
|
|
old_init = CreditCardForm.__init__
|
|
|
|
@wraps(old_init)
|
|
def new_init(self, *a, **k):
|
|
|
|
# Map the names from our attendee profile model
|
|
# To the values expected in the Stripe card model
|
|
mappings = (
|
|
("address_line_1", "address_line1"),
|
|
("address_line_2", "address_line2"),
|
|
("address_suburb", "address_city"),
|
|
("address_postcode", "address_zip"),
|
|
("state", "address_state"),
|
|
("country", "address_country"),
|
|
)
|
|
|
|
initial = "initial"
|
|
if initial not in k:
|
|
k[initial] = {}
|
|
initial = k[initial]
|
|
|
|
# Find request context maybe?
|
|
frame = inspect.currentframe()
|
|
attendee_profile = None
|
|
if frame:
|
|
context = frame.f_back.f_locals
|
|
for name, value in (context.items() or {}):
|
|
if not isinstance(value, HttpRequest):
|
|
continue
|
|
user = value.user
|
|
if not user.is_authenticated:
|
|
break
|
|
try:
|
|
attendee_profile = models.AttendeeProfile.objects.get(
|
|
attendee__user=user
|
|
)
|
|
except models.AttendeeProfile.DoesNotExist:
|
|
# Profile is still none.
|
|
pass
|
|
break
|
|
|
|
if attendee_profile:
|
|
for us, stripe in mappings:
|
|
i = getattr(attendee_profile, us, None)
|
|
if i:
|
|
initial[stripe] = i
|
|
|
|
old_init(self, *a, **k)
|
|
|
|
CreditCardForm.__init__ = new_init
|