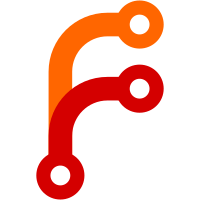
In many parts of the schedule there are multiple slots with the same start/end times, and it can be hard to find the one you want to edit. Make this slightly simpler by listing the room names in the admin list.
71 lines
1.8 KiB
Python
71 lines
1.8 KiB
Python
from django.contrib import admin
|
|
|
|
from symposion.schedule.models import Schedule, Day, Room, SlotKind, Slot, SlotRoom, Presentation, Session, SessionRole, Track
|
|
|
|
|
|
class DayInline(admin.StackedInline):
|
|
model = Day
|
|
extra = 2
|
|
|
|
|
|
class SlotKindInline(admin.StackedInline):
|
|
model = SlotKind
|
|
|
|
|
|
class ScheduleAdmin(admin.ModelAdmin):
|
|
model = Schedule
|
|
inlines = [DayInline, SlotKindInline, ]
|
|
list_display = ["section","published","hidden"]
|
|
list_editable = ["published","hidden"]
|
|
list_filter = ["published","hidden"]
|
|
|
|
|
|
class SlotRoomInline(admin.TabularInline):
|
|
model = SlotRoom
|
|
extra = 1
|
|
|
|
|
|
class SlotAdmin(admin.ModelAdmin):
|
|
list_filter = ("day", "kind","exclusive")
|
|
list_display = ("day", "start", "end", "kind", "room_names",
|
|
"content_override","exclusive")
|
|
list_editable = ("exclusive",)
|
|
inlines = [SlotRoomInline]
|
|
|
|
def room_names(self, slot):
|
|
return ", ".join(room.name for room in slot.rooms.all())
|
|
|
|
|
|
class RoomAdmin(admin.ModelAdmin):
|
|
list_display = ["name", "order", "schedule"]
|
|
list_filter = ["schedule"]
|
|
inlines = [SlotRoomInline]
|
|
|
|
|
|
class PresentationAdmin(admin.ModelAdmin):
|
|
model = Presentation
|
|
list_filter = ("section", "cancelled", "slot")
|
|
|
|
class TrackAdmin(admin.ModelAdmin):
|
|
model = Track
|
|
list_filter=("room","day")
|
|
list_display=("name","room","day")
|
|
list_editable=("room","day")
|
|
|
|
|
|
admin.site.register(Day)
|
|
admin.site.register(
|
|
SlotKind,
|
|
list_display=["label", "schedule"],
|
|
)
|
|
admin.site.register(
|
|
SlotRoom,
|
|
list_display=["slot", "room"]
|
|
)
|
|
admin.site.register(Schedule, ScheduleAdmin)
|
|
admin.site.register(Room, RoomAdmin)
|
|
admin.site.register(Slot, SlotAdmin)
|
|
admin.site.register(Session)
|
|
admin.site.register(SessionRole)
|
|
admin.site.register(Presentation, PresentationAdmin)
|
|
admin.site.register(Track, TrackAdmin)
|