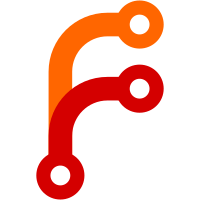
I cannot find the benifit to this over the base editor. Tabs work, but that's pretty minimal. And tabs don't work on GitLab or GitHub, so I don't feel not having that is substantual to functionality.
64 lines
1.5 KiB
Python
64 lines
1.5 KiB
Python
from django import forms
|
|
|
|
from pinaxcon.proposals.models import TalkProposal, TutorialProposal, MiniconfProposal
|
|
|
|
|
|
class ProposalForm(forms.ModelForm):
|
|
|
|
required_css_class = 'label-required'
|
|
|
|
def clean_description(self):
|
|
value = self.cleaned_data["description"]
|
|
if len(value) > 400:
|
|
raise forms.ValidationError(
|
|
u"The description must be less than 400 characters"
|
|
)
|
|
return value
|
|
|
|
|
|
class TalkProposalForm(ProposalForm):
|
|
|
|
class Meta:
|
|
model = TalkProposal
|
|
fields = [
|
|
"title",
|
|
"target_audience",
|
|
"abstract",
|
|
"private_abstract",
|
|
"technical_requirements",
|
|
"project",
|
|
"project_url",
|
|
"video_url",
|
|
"recording_release",
|
|
"materials_release",
|
|
]
|
|
|
|
|
|
class TutorialProposalForm(ProposalForm):
|
|
|
|
class Meta:
|
|
model = TutorialProposal
|
|
fields = [
|
|
"title",
|
|
"target_audience",
|
|
"abstract",
|
|
"private_abstract",
|
|
"technical_requirements",
|
|
"project",
|
|
"project_url",
|
|
"video_url",
|
|
"recording_release",
|
|
"materials_release",
|
|
]
|
|
|
|
|
|
class MiniconfProposalForm(ProposalForm):
|
|
|
|
class Meta:
|
|
model = MiniconfProposal
|
|
fields = [
|
|
"title",
|
|
"abstract",
|
|
"private_abstract",
|
|
"technical_requirements",
|
|
]
|