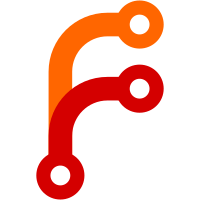
Update dates and descriptions for LCA2022. Remove old miniconf proposal types. Add local timezone to speaker profile.
87 lines
2.4 KiB
Python
87 lines
2.4 KiB
Python
from django.db import models
|
|
|
|
from symposion.proposals.models import ProposalBase
|
|
|
|
|
|
class Proposal(ProposalBase):
|
|
|
|
TARGET_USER = 1
|
|
TARGET_BUSINESS = 2
|
|
TARGET_COMMUNITY = 3
|
|
TARGET_DEVELOPER = 4
|
|
|
|
TARGET_AUDIENCES = [
|
|
(TARGET_USER, "User"),
|
|
(TARGET_BUSINESS, "Business"),
|
|
(TARGET_COMMUNITY, "Community"),
|
|
(TARGET_DEVELOPER, "Developer"),
|
|
]
|
|
|
|
target_audience = models.IntegerField(choices=TARGET_AUDIENCES)
|
|
|
|
recording_release = models.BooleanField(
|
|
default=True,
|
|
help_text="I allow Linux Australia to release any recordings of "
|
|
"presentations covered by this proposal, under the <a "
|
|
"href='https://creativecommons.org/licenses/by-sa/3.0/au/deed.en'> "
|
|
"Creative Commons Attribution-Share Alike Australia 3.0 Licence</a>"
|
|
)
|
|
|
|
materials_release = models.BooleanField(
|
|
default=True,
|
|
help_text="I allow Linux Australia to release any other material "
|
|
"(such as slides) from presentations covered by this proposal, under "
|
|
"the <a "
|
|
"href='https://creativecommons.org/licenses/by-sa/3.0/au/deed.en'> "
|
|
"Creative Commons Attribution-Share Alike Australia 3.0 Licence</a>"
|
|
)
|
|
|
|
class Meta:
|
|
abstract = True
|
|
|
|
|
|
class TalkProposal(Proposal):
|
|
|
|
class Meta:
|
|
verbose_name = "talk proposal"
|
|
|
|
|
|
class TutorialProposal(Proposal):
|
|
|
|
class Meta:
|
|
verbose_name = "tutorial proposal"
|
|
|
|
|
|
class MiniconfProposal(Proposal):
|
|
|
|
target_audience = models.IntegerField(choices=Proposal.TARGET_AUDIENCES,
|
|
default=Proposal.TARGET_DEVELOPER)
|
|
|
|
class Meta:
|
|
verbose_name = "miniconf proposal"
|
|
|
|
|
|
class MiniconfSessionProposal(Proposal):
|
|
|
|
FORMAT_SHORT_PRESENTATION = 1
|
|
FORMAT_LONG_PRESENTATION = 2
|
|
|
|
TALK_FORMATS = [
|
|
(FORMAT_SHORT_PRESENTATION, "Short Presentation (15 or 20 min)"),
|
|
(FORMAT_LONG_PRESENTATION, "Long Presentation (45 min)"),
|
|
]
|
|
|
|
talk_format = models.IntegerField(
|
|
choices=TALK_FORMATS,
|
|
default=FORMAT_LONG_PRESENTATION,
|
|
help_text="Please indicate your preferred talk length in the private abstract field below."
|
|
)
|
|
|
|
ticket_acknowledgement = models.BooleanField(
|
|
default=False,
|
|
help_text="I understand that I may be required to purchase a conference ticket "
|
|
"as linux.conf.au miniconfs are unfunded community run events."
|
|
)
|
|
|
|
class Meta:
|
|
abstract = True
|