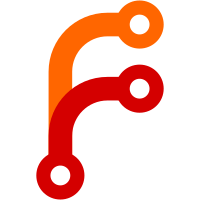
These are various changes to support the changes to the postal address table to include split-out fields for parts of the address instead of just the formatted_address field. The changes herein are not comprehensive to solve all the problems and issues associated with this update, but they are enough changes to get most daily operations with the database more-or-less working.
115 lines
4.4 KiB
Perl
Executable file
115 lines
4.4 KiB
Perl
Executable file
#!/usr/bin/perl
|
|
|
|
use strict;
|
|
use warnings;
|
|
use utf8;
|
|
|
|
use autodie qw(open close);
|
|
use DBI;
|
|
|
|
binmode STDOUT, ":utf8";
|
|
binmode STDIN, ":utf8";
|
|
|
|
use Supporters;
|
|
|
|
if (@ARGV < 2) {
|
|
print STDERR "usage: $0 <SUPPORTERS_SQLITE_DB_FILE> <CRITERION> <SEARCH_PARAMETER> <VERBOSE>\n";
|
|
exit 1;
|
|
}
|
|
|
|
my($SUPPORTERS_SQLITE_DB_FILE, $CRITERION, $SEARCH_PARAMETER, $VERBOSE) = @ARGV;
|
|
$VERBOSE = 0 if not defined $VERBOSE;
|
|
|
|
my $dbh = DBI->connect("dbi:SQLite:dbname=$SUPPORTERS_SQLITE_DB_FILE", "", "",
|
|
{ RaiseError => 1, sqlite_unicode => 1 })
|
|
or die $DBI::errstr;
|
|
|
|
my $sp = new Supporters($dbh, ['none']);
|
|
|
|
my $found = 0;
|
|
my(@supporterIds);
|
|
die "Can only search on id, ledgerEntityId, emailAddress" unless $CRITERION =~ /^(ledgerEntityId|emailAddress|id)$/;
|
|
if ($CRITERION eq 'id') {
|
|
push(@supporterIds, $SEARCH_PARAMETER);
|
|
} else {
|
|
@supporterIds = $sp->findDonor({$CRITERION => $SEARCH_PARAMETER });
|
|
|
|
}
|
|
my @requestTypes = $sp->getRequestType();
|
|
foreach my $id (@supporterIds) {
|
|
$found = 1;
|
|
my $rtTicket = $sp->_getDonorField('rt_ticket', $id);
|
|
$rtTicket = "" if not defined $rtTicket;
|
|
$rtTicket = "[sfconservancy.org #$rtTicket]" if $rtTicket ne "";
|
|
my $preferredEmail = $sp->getPreferredEmailAddress($id);
|
|
my $preferredPostal = $sp->getPreferredPostalAddress($id);
|
|
print "Found: BEGIN: $id, ", $sp->getLedgerEntityId($id), "\n";
|
|
print " RT: $rtTicket" if $rtTicket ne "";
|
|
print " Public Ack: ";
|
|
if (not defined $sp->getPublicAck($id)) {
|
|
print "unknown\n";
|
|
} elsif ($sp->getPublicAck($id)) {
|
|
print "yes\n";
|
|
print " Display name for public ack: \"", $sp->getDisplayName($id), "\"\n";
|
|
} else {
|
|
print "no\n";
|
|
}
|
|
my(%addr) = $sp->getEmailAddresses($id);
|
|
print " Email Addresses: ";
|
|
my $cnt = 0;
|
|
foreach my $email (keys %addr) {
|
|
$cnt++;
|
|
print $email;
|
|
print "(preferred)" if (defined $preferredEmail) and $email eq $preferredEmail;
|
|
print ", " unless $cnt == scalar keys %addr;
|
|
}
|
|
my(%postalAddresses) = $sp->getPostalAddresses($id);
|
|
if (scalar keys %postalAddresses <= 0) {
|
|
print "\n NO POSTAL ADDRESSES.\n";
|
|
} else {
|
|
print "\n Postal Addresses:\n";
|
|
foreach my $addrID (sort
|
|
{ $postalAddresses{$a}{date_valid_from} cmp $postalAddresses{$b}{date_valid_from} or
|
|
$postalAddresses{$a}{date_valid_to} cmp $postalAddresses{$b}{date_valid_to};
|
|
} keys %postalAddresses) {
|
|
print " Address $addrID, found in $postalAddresses{$addrID}{type}, valid from $postalAddresses{$addrID}{date_valid_from} until ",
|
|
(not defined $postalAddresses{$addrID}{date_valid_to}) ? "RIGHT NOW " : $postalAddresses{$addrID}{date_valid_to}, ":\n";
|
|
foreach my $key (qw/first_name middle_name last_name organization address_1 address_2 address_3 city
|
|
state_province_or_region postcode country/) {
|
|
print " $key: $postalAddresses{$addrID}{$key}\n" if (defined $postalAddresses{$addrID}{$key});
|
|
}
|
|
}
|
|
}
|
|
foreach my $requestType (@requestTypes) {
|
|
my $req = $sp->getRequest({ donorId => $id, requestType => $requestType});
|
|
if (defined $req) {
|
|
print " Request $req->{requestId} of $req->{requestType}";
|
|
print "($req->{requestConfiguration})" if defined $req->{requestConfiguration};
|
|
print " made on $req->{requestDate}";
|
|
if (defined $req->{fulfillDate}) {
|
|
print "...\n fulfilled on $req->{fulfillDate}";
|
|
print "...\n by: $req->{fulfilledBy}" if defined $req->{fulfilledBy};
|
|
print "...\n via: $req->{fulfilledVia}" if defined $req->{fulfilledVia};
|
|
}
|
|
if (defined $req->{holdDate} ) {
|
|
print "...\n put on hold on $req->{holdDate} by $req->{holder}";
|
|
print "...\n release on: $req->{holdReleaseDate}" if defined $req->{holdReleaseDate};
|
|
print "...\n on hold because: $req->{heldBecause}" if defined $req->{heldBecause};
|
|
}
|
|
if (defined $req->{notes}) {
|
|
print "...\n Notes: $req->{notes}";
|
|
}
|
|
print "\n\n";
|
|
}
|
|
}
|
|
print "END: $id, ", $sp->getLedgerEntityId($id);
|
|
print " RT: $rtTicket" if $rtTicket ne "";
|
|
print "\n";
|
|
}
|
|
print "No entries found\n" unless $found;
|
|
###############################################################################
|
|
#
|
|
# Local variables:
|
|
# compile-command: "perl -I../Supporters/lib -c find-supporter.plx"
|
|
# End:
|
|
|